In my last post I created a twitter developer account. In this post I will access Twitter with a Java program.
Setup Eclipse Project
Create a new Maven Project:
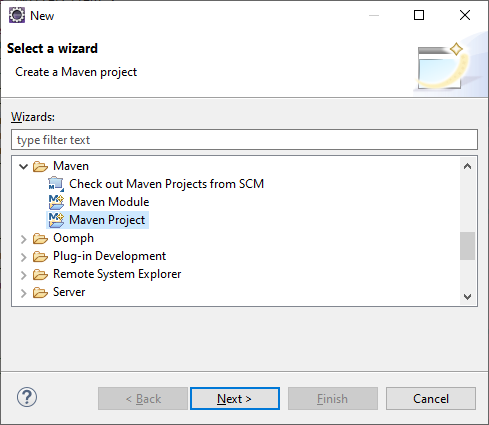
Create a simple project (skip archetype selection).
Change JRE System Library from Java 1.5 to Java 1.8.
Add Twitter4J to Maven dependencies:
<project xmlns="http://maven.apache.org/POM/4.0.0" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xsi:schemaLocation="http://maven.apache.org/POM/4.0.0 http://maven.apache.org/xsd/maven-4.0.0.xsd"> <modelVersion>4.0.0</modelVersion> <groupId>de.kaulbach</groupId> <artifactId>twitter</artifactId> <version>1.0-SNAPSHOT</version> <packaging>jar</packaging> <properties> <maven.compiler.source>1.8</maven.compiler.source> <maven.compiler.target>1.8</maven.compiler.target> <project.build.sourceEncoding>UTF-8</project.build.sourceEncoding> <project.reporting.outputEncoding>UTF-8</project.reporting.outputEncoding> </properties> <dependencies> <dependency> <groupId>org.twitter4j</groupId> <artifactId>twitter4j-stream</artifactId> <version>4.0.7</version> </dependency> </dependencies> <build> <finalName>twitter</finalName> </build> </project>
Configuration
Create a new file twitter4j.properties in folder src/main/resources with account properties from developer console:
oauth.consumerKey = // your key (API key) oauth.consumerSecret = // your secret (API secret key) oauth.accessToken = // your token oauth.accessTokenSecret = // your token secret
There are some other ways to configure (Java Code, Environment Variables, System Properties), see here
Java Code
Write a simple Code Example to show 7 Tweets with HashTag #Happy:
public class TwitterClient { public static void main(String[] args) throws Exception { Twitter twitter = TwitterFactory.getSingleton(); Query query = new Query("#Happy"); query.setCount(7); QueryResult result = twitter.search(query); for (Status status : result.getTweets()) { System.out.println("--"); System.out.println("@" + status.getUser().getScreenName() + ":" + status.getText()); } System.out.println("----------------------------------------"); } }
Change code to show Tweets of Pope Francis with God in it:
Query query = new Query("from:Pontifex God");